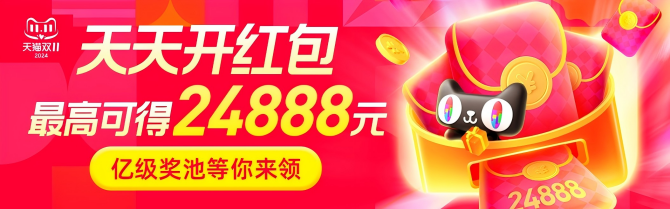
NTL
官网:https://libntl.org/doc/tour.html
NTL is a high-performance, portable C++ library providing data structures and algorithms for arbitrary length integers; for vectors, matrices, and polynomials over the integers and over finite fields; and for arbitrary precision floating point arithmetic.
NTL provides high quality implementations of state-of-the-art algorithms for:
- arbitrary length integer arithmetic and arbitrary precision floating point arithmetic;
- polynomial arithmetic over the integers and finite fields including basic arithmetic, polynomial factorization, irreducibility testing, computation of minimal polynomials, traces, norms, and more;
- lattice basis reduction, including very robust and fast implementations of Schnorr-Euchner, block Korkin-Zolotarev reduction, and the new Schnorr-Horner pruning heuristic for block Korkin-Zolotarev;
- basic linear algebra over the integers, finite fields, and arbitrary precision floating point numbers.
类型介绍
The basic ring classes are:
ZZ
: big integersZZ_p
: big integers modulo pzz_p
: integers mod “single precision” pGF2
: integers mod 2ZZX
: univariate polynomials over ZZZZ_pX
: univariate polynomials over ZZ_pzz_pX
: univariate polynomials over zz_pGF2X
: polynomials over GF2ZZ_pE
: ring/field extension over ZZ_pzz_pE
: ring/field extension over zz_pGF2E
: ring/field extension over GF2ZZ_pEX
: univariate polynomials over ZZ_pEzz_pEX
: univariate polynomials over zz_pEGF2EX
: univariate polynomials over GF2E
使用
- 常用函数
SetSeed(const ZZ& s)
:设置PRF种子
RandomBnd(ZZ& x, const ZZ& n)
:x∈{0,1,⋯n−1}x \in \{0,1,\cdots n-1\}x∈{0,1,⋯n−1},如果 n≤0n \le 0n≤0 那么 x=0x=0x=0
RandomBits(ZZ& x, long l)
:随机生成lll比特的整数
ZZ p(17)
:初始化整数为17,这里参数类型是long
p = to_ZZ("123")
:读入字符串,可输入大整数
GenPrime(p, 8)
:随机生成8比特素数
ZZ_p::init(p)
:初始化环ZpZ_pZp
ZZ_p a(2)
:初始化为 2modp2 \mod p2modp,这里参数类型是long
random(a)
:随机生成ZpZ_pZp中元素
ZZ_pX m
:Zp[x]Z_p[x]Zp[x]中的多项式,记录为向量ZpnZ_p^nZpn
SetCoeff(m, 5)
:将x5x^5x5系数置为 1
m[0]=1
:将x0x^0x0系数置为 1
BuildIrred(m, 3)
:随机生成3次不可约多项式
ZZ_pE::init(m)
:初始化环Zp[x]/(m(x))Z_p[x]/(m(x))Zp[x]/(m(x)),若ppp是素数且m(x)m(x)m(x)是d次不可约多项式,那么它同构于有限域GF(pd)GF(p^d)GF(pd)
ZZ_pEX f, g, h
:GF(pd)[x]GF(p^d)[x]GF(pd)[x]上的多项式,记录为向量GF(pd)nGF(p^d)^nGF(pd)n
random(f, 5)
:随机生成5次多项式
h = sqr(g) % f
:计算h≡g2modfh \equiv g^2 \mod fh≡g2modf
- 环GF(pd)[x]/(xn−1)GF(p^d)[x]/(x^n-1)GF(pd)[x]/(xn−1)上多项式运算:
#include <iostream>#include <NTL/ZZ_p.h> // integers mod p
#include <NTL/ZZ_pX.h> // polynomials over ZZ_p
#include <NTL/ZZ_pE.h> // ring/field extension of ZZ_p
#include <NTL/ZZ_pEX.h> // polynomials over ZZ_pE
#include <NTL/ZZ_pXFactoring.h>
#include <NTL/ZZ_pEXFactoring.h>using namespace std;
using namespace NTL;#pragma comment(lib, "NTL")int main()
{ZZ p(17); //初始化为17//群Z_pZZ_p::init(p); //随机生成Z_p[x]中的d次不可约多项式int d = 4;ZZ_pX m;BuildIrred(m, d); //域GF(p^d) = Z_p[x]/m(x)ZZ_pE::init(m); //GF(p^d)[x]中的多项式ZZ_pEX f, g, h; // f(x) = x^8 - 1SetCoeff(f, 8); //将 x^8 系数置为 1SetCoeff(f, 0, -1); //将 x^0 系数置为 -1//随机生成5次多项式random(g, 5);// 环上多项式的运算:h = g^2 mod fh = sqr(g) % f; cout << "p = " << p << endl;cout << "d = " << d << endl;cout << "m(x) = " << m << endl;cout << "f = " << f << endl;cout << "g = " << g << endl;cout << "h = " << h << endl;return 0;
}