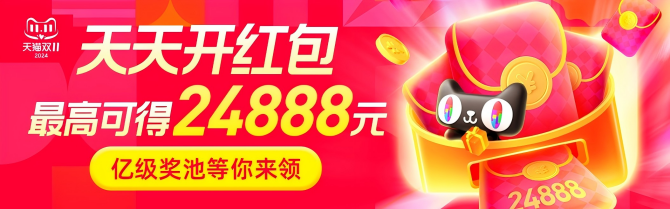
列表
image.png
image.png
代码实现
List = ['Objective-c','Swift','Python','Java','C++','C']
print('List now content:',List)
print('List length is',len(List))
for item in List:
print('List content: ',item,end=' \n')
List.append('Ruby')//追加元素
print('List now content:',List)
List.sort()//排序
print('List sort content:',List)
print('List first item:',List[0])
firstItem = List[0]
del List[0]//删除元素
print('firstItem is:',firstItem)
print('List finally is:',List)
运行结果
List now content: ['Objective-c', 'Swift', 'Python', 'Java', 'C++', 'C']
List length is 6
List content: Objective-c
List content: Swift
List content: Python
List content: Java
List content: C++
List content: C
List now content: ['Objective-c', 'Swift', 'Python', 'Java', 'C++', 'C', 'Ruby']
List sort content: ['C', 'C++', 'Java', 'Objective-c', 'Python', 'Ruby', 'Swift']
List first item: C
firstItem is: C
List finally is: ['C++', 'Java', 'Objective-c', 'Python', 'Ruby', 'Swift']
列表是可变的,字符串是不可变的
元组 Tuple
元组是不可变的,不可以编辑或更改元组
image.png
代码实现
mouth = ('day 1','day 2','day 3','day 4','day 5')
print('mouth length is :',len(mouth))
new_mouth = mouth,'day 11','day'
print('new mouth is :',new_mouth)
print('old mouth is :',new_mouth[0])
print('last item of old mouth is :',new_mouth[0][len(mouth)-1])
print('new mouth length is :',len(new_mouth))
运行结果
mouth length is : 5
new mouth is : (('day 1', 'day 2', 'day 3', 'day 4', 'day 5'), 'day 11', 'day')
old mouth is : ('day 1', 'day 2', 'day 3', 'day 4', 'day 5')
last item of old mouth is : day 5
new mouth length is : 3
new mouth actually length is 7
image.png
包含一个元素的元组
mouth = ('day1', )//必须在第一个元素后面加逗号,以便程序区分
字典
键值对,key-value。key 只能是不可变得对象,value 是可变和不可变都可以。
样式:dict = {name:joy,age:18}
示例代码
student = {
'name' : 'joy',
'age' : 18,
'stuNo': '036',
'birth':'1994-11-24',
}
print('student message is:',student)
print('student name is:',student['name'])
del student['birth']
print('now student message is {}'.format(student))
for name, age in student.items():
print('name:{} and age :{}'.format(name,age))
student['place'] = 'BeiJing'
if 'place' in student:
print('now student message is {}'.format(student))
输出结果
student message is: {'name': 'joy', 'age': 18, 'stuNo': '036', 'birth': '1994-11-24'}
student name is: joy
now student message is {'name': 'joy', 'age': 18, 'stuNo': '036'}
name:name and age :joy
name:age and age :18
name:stuNo and age :036
now student message is {'name': 'joy', 'age': 18, 'stuNo': '036', 'place': 'BeiJing'}
image.png
序列
image.png
代码实现
List = ['Objective-c','Swift','Python','Java','C++','C']
new_item = 'single Language'
print('item 0 is:',List[0])
print('item 3 is:',List[3])
print('item -2 is:',List[-2])
print('item -1 is:',List[-1])
print('new_item is:',new_item[7])
print('===========================')
# 列表截取
print('item 1-3:',List[1:3])
print('item 2-end is:',List[2:])
print('item 1to-1 is:',List[1:-1])
print('item 0-end is:',List[:])
# 字符串截取
print('new_item 7-end is:',new_item[7:])
print('item 0-4:',new_item[0:4])
print('item 4to-4:',new_item[4:-4])
输出结果
item 0 is: Objective-c
item 3 is: Java
item -2 is: C++
item -1 is: C
new_item is: L
===========================
item 1-3: ['Swift', 'Python']
item 2-end is: ['Python', 'Java', 'C++', 'C']
item 1to-1 is: ['Swift', 'Python', 'Java', 'C++']
item 0-end is: ['Objective-c', 'Swift', 'Python', 'Java', 'C++', 'C']
new_item 7-end is: Language
item 0-4: sing
item 4to-4: le Lang
使用索引获取序列中各个项目,“[ ]”中指定序列的制定数组,Python 将返回该序列制定位置的项目,Python计数从0开始。索引计数也可以为负数,负数表示从序列的末尾向首位开始计数。
image.png
还可以设置截取跨度长度
List[ : : 2]//表示从起始-结尾 截取长度为2的项目
image.png
集合
代码实现
stu = set(['jone','tom','jerry','jack'])
print('stu:',stu)
print('jack is in stu? ','jack' in stu)
print('lee is in stu? ','lee' in stu)
stus = stu.copy()
stus.add('jodan')
print('stus:',stus)
print('stus is stu superset? ',stus.issuperset(stu))
print('stus and stu all item? ',stu | stus)
stus.remove('tom')
stus.add('robert')
print('stu:',stu)
print('stus:',stus)
print('stus and stu all item? ',stu | stus)
print('stus and stu intersection item? ',stu & stus)
输出结果
stu: {'jack', 'tom', 'jerry', 'jone'}
jack is in stu? True
lee is in stu? False
stus: {'tom', 'jack', 'jerry', 'jodan', 'jone'}
stus is stu superset? True
stus and stu all item? {'tom', 'jack', 'jerry', 'jodan', 'jone'}
stu: {'jack', 'tom', 'jerry', 'jone'}
stus: {'jack', 'robert', 'jerry', 'jodan', 'jone'}
stus and stu all item? {'tom', 'jack', 'robert', 'jerry', 'jodan', 'jone'}
stus and stu intersection item? {'jack', 'jerry', 'jone'}
set 支持拷贝、增加、删除等基本操作。
引用
创建一个对象,分配给该对象某个变量,变量只会 refer 某个对象,不会代表对象本身。变量名只是指向计算机内存储该对象的内存地址。在引用时候会出现不同的效果。
案例
List = ['Objective-c','Swift','Python','Java','C++','C']
new_list = List
# new_list 只是指向 List 地址的名称,List 改变,new_list也会随之改变
List.remove("C")
print('List:',List)
print('newList:',new_list)
# new_list 与 List 指向同一对象
copy_list = List[:]
# 通过切片 复制出一份 List 的副本
print('copy_list:',copy_list)
del copy_list[0]
print('List:',List)
print('newList:',new_list)
print('copy_list:',copy_list)
输出结果
List: ['Objective-c', 'Swift', 'Python', 'Java', 'C++']
newList: ['Objective-c', 'Swift', 'Python', 'Java', 'C++']
copy_list: ['Objective-c', 'Swift', 'Python', 'Java', 'C++']
List: ['Objective-c', 'Swift', 'Python', 'Java', 'C++']
newList: ['Objective-c', 'Swift', 'Python', 'Java', 'C++']
copy_list: ['Swift', 'Python', 'Java', 'C++']
如果想操作而又不影响源数据的情况下,使用 “ List[ : ] ” 这样得到的序列是拷贝出一份副本,互相操作不会影响;如果采用 “ a=b ”,则操作会造成联动,慎用!
字符串补充
代码实现
List = ['Objective-c','Swift','Python','Java','C++','C']
str = 'python'
if str.startswith('py'):
# 字符串以什么开头
print('str start at \'py\'')
if 'on' in str:
# 字符串包含
print('str contain \'on\'')
if str.find('th') != -1:
# 查找字符串的位置,如果找不到则返回"-1"
print('\'th\' in str location is:',str.find('th'))
otherStr = ' ~ '
print('List change str is:',otherStr.join(List))
#otherStr 是作为List 中每个项目的分隔符,返回一个更大的字符串
输出结果
str start at 'py'
str contain 'on'
'th' in str location is: 2
List change str is: Objective-c ~ Swift ~ Python ~ Java ~ C++ ~ C