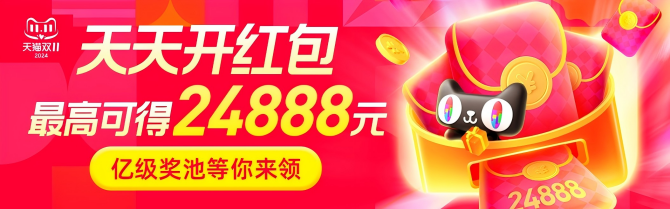
# coding: UTF-8 import os import sys import salt.client import salt.config import time from ftplib import FTP, FTP_TLS import json from subprocess import Popen, PIPE, STDOUT agent_ids = agent_ip.split(',') if '{' in sys_type: sys_types=json.loads(sys_type) else: sys_types={} for i in agent_ids: sys_types.update({i:sys_type}) def get_source_file_path(source_file_path,agent_id): global sys_types sys_type=sys_types.get(agent_id) if sys_type == 'windows': if source_file_path.startswith('/'): source_file_path='c:'+source_file_path source_file_path = source_file_path.replace('\\', '/') source_file_path = source_file_path.replace('\', '/') source_file_path=source_file_path.decode('gbk') print source_file_path else: if isinstance(source_file_path, unicode): source_file_path = source_file_path.encode('utf8') file_paths = source_file_path.split(' ') return file_paths if isinstance(ftp_dir, unicode): ftp_dir = ftp_dir.encode('utf8') BASE_URL = os.getenv('ANT_BASEURL') fail = [] success = [] def agent_to_proxy(): global agent_ids, source_file_path, success, fail, BASE_URL proxy_path = os.getenv('ANT_MODULE_ROOT', '') master_conf = os.path.join(proxy_path, 'conf', 'master') if not os.path.exists(master_conf): print('Proxy 路径不正确 {}'.format(proxy_path)) sys.exit(1) opts = salt.config.client_config(master_conf) LocalClient = salt.client.LocalClient(master_conf) CACHE_DIR = opts['cachedir'] for agent_id in agent_ids: file_paths=get_source_file_path(source_file_path, agent_id) print file_paths ret = LocalClient.cmd(agent_id, 'cp.push', file_paths, expr_form='list') no_exist_hosts = set(ret.keys()) - set(agent_ids) print no_exist_hosts if no_exist_hosts: print('异常:{} agent 不存在'.format(list(no_exist_hosts))) sys.exit(1) for file_path in file_paths: for host, status in ret.iteritems(): if status is True: proxy_file_path = os.path.join(CACHE_DIR, 'minions', host, 'files', file_path.lstrip('/')) res = proxy_to_ftp(proxy_file_path) if res: print file_path, '上传成功' else: fail.append('{}:{}'.format(host, file_path)) elif status is False: print('{}:{} 上传失败,可能文件不存在'.format(host, file_path)) fail.append('{}:{}'.format(host, file_path)) else: print('异常:{}:{} 上传失败,{}'.format(host, file_path, status)) fail.append('{}:{}'.format(host, file_path)) def _conn_ftp(user, passwd): global ftp_host, ftp_port, connect_type conn = False ispasv = False if user in (None, 'false') else True ftp_url = ftp_host connect_type = connect_type.lower() port = ftp_port try: if ':' in ftp_url: conn = True ftp_url, port = ftp_url.split(':') if conn: ftp = FTP() ftp.connect(host=str(ftp_url), port=int(port)) else: ftp = FTP(ftp_url) ftp.login(user, passwd) except Exception as e: if 'requires SSL.' in '{}'.format(e): try: if conn: ftp = FTP_TLS() ftp.connect(host=ftp_url, port=int(port)) else: ftp = FTP_TLS(ftp_url) ftp.login(user, passwd) except Exception as e: print e sys.exit(1) else: print e sys.exit(1) return ftp, ispasv def proxy_to_ftp(proxy_file_path): global username, password, ftp_dir ftp, ispasv = _conn_ftp(username, password) ftp.encoding = "utf-8" try: ftp.set_pasv(ispasv) # ftp.retrlines('LIST') except: ftp.set_pasv(not ispasv) now_path = ftp.pwd() print now_path if ftp_dir and ftp_dir != '/': try: ftp.cwd(ftp_dir) except: new_path = os.path.join(now_path, ftp_dir.lstrip('/')) print new_path ftp.mkd(new_path) ftp.cwd(new_path) try: ftp.rename(os.path.basename(proxy_file_path).decode("utf8").encode('gbk'), os.path.basename(proxy_file_path).decode("utf8").encode('gbk')+'.'+time.strftime('%Y-%m-%d_%H:%M:%S')) except: pass print proxy_file_path with open(proxy_file_path, 'rb') as f_: result = ftp.storbinary('STOR %s' % os.path.basename(proxy_file_path).decode("utf8").encode('gbk'), f_) ftp.close() if 'Transfer complete.' in result: return True else: return False try: agent_to_proxy() if success: print '文件传输成功:{}'.format(','.join(success)) if fail: print '文件传输失败:{}'.format(','.join(fail)) sys.exit(1) except Exception as e: print e sys.exit(1)